Components - Android Development
Basics
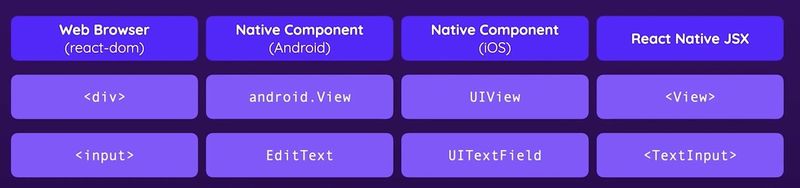
<View>
=> Uses flexbox by default
<SafeAreaView>
=> Gives default padding from the top, Only of iOS
<Text>
numberOfLines={n}
=> Truncates the texts exceeding this
onPress={functionName}
=> Like onClick()
<Image>
source={require("filePath")}
=> Returns a number that is reference to the local image
source={{width: 200, height: 300, uri: "imageURL",}}
=> For network image
blurRadius={1}
fadeDuration={1000}
=> Fade effect on start, Only for Android
resizeMode = "contain"
<TextInput>
<StyleSheet>
- Basic
- Doesn't have cascading effect
- Standard
style={variable}
style={{ propertyName: value}}
style={styles.variable}
- When using
const styles = StyleSheet.create({})
, returns a Object
- Validates the properties unlike plain JS object
style={[styles.variable1, styles.variable2]}
- Properties of right overwrites the left
<Touchable>
<TouchableWithoutFeedback>
=> Wrap components with this
onPress={functionName}
=> Like onClick()
<TouchableOpacity>
=> Like TouchableWithoutFeedback but reduces opacity for a moment when clicked
<TouchableHighlight>
=> Like TouchableWithoutFeedback but darkens background for a moment when clicked
<Pressable>
onPress
android_ripple
=> Style for Android
style
=> Style for IOS
<Modal>
- UI
<Button>
<Picker>
<Slider>
<Switch>
- List Views
<ScrollView>
=> Adds Scroll bar when required
<FlatList>
=> Lazy loading
data
=> Array/Object
renderItem
=> Returns items
keyExtractor
=> If "key" named key is not present in the array object
<SelectionList>
- IOS
<ActionSheetIOS>
<AlertIOS>
- Android
<BackHandler>
<DatePickerAndroid>
API
Alert
alert("Message")
=> Standard
Alert.alert("Title", "Message", [{text: "button1"}, {text: "button2", onPress: functionName}])
Alert.prompt("Title", "Message", functionName)
=> Only for iOS
Platform
=> For writing platform specific style
StatusBar
Dimensions
=> Numbers like "n" are Density Independent Pixels (DIP) => Physical Pixel = DIP x Scale Factor => Phone Pixel = Points x Scale Factor
- get() => Takes the value "window" & "screen", which is same for iOS, window size is smaller for Android
Others
- Vector Icons
npm i react-native-vector-icons
npx react-native link react-native-vector-icons